First Steps with the codePost Python SDK
This tutorial describes how to install the codePost Python SDK, retrieve your API key from the codePost user settings page, and show how to make your first call to the codePost API—to retrieve a list of the courses you have access to.
While codePost is a useful tool on its own, combined with the codePost API, it is fairly unique in that it provides you with the limitless ability to automate repetitive tasks that you used to be doing, but also to automate repetitive tasks that you never considered doing and which may help unlock interesting insights.
You will interact with codePost through the same API (Application Programming Interface) that we use ourselves, thus you will be able to programmatically do anything that can be done on codePost. In this tutorial, we will show how to use the Python language SDK. Python is our favorite language, and the one with which we designed the codePost server.
We hope to provide language-specific codePost SDKs in the near future. If you have a favorite language you would like us to prioritize, please shoot us an email at [email protected]!
Before getting started: Your Python environment
You will need to have a recent distribution of Python installed on your computer: While Python 2.7 is supported, we recommend if possible using Python 3.5 or more recent. Many operating systems nowadays come with Python pre-installed. You can check if yours does too by opening a terminal and typing python -V
. Next, you can type pip -V
, to check whether you have the Python package manager which comes as part of Python 3.x versions of the language, but needs to be installed separately in earlier versions.
Finally, if you would prefer to follow entirely online, you can go to Repl.it, install the codepost
package using the left sidebar's appropriate tab.
More information on setting up your Python environment can be found at The Hitchhiker's Guide to Python or RealPython.
1. Installing the codePost Python SDK
The codePost Python SDK is published on PyPi, which is Python's dedicated package management system. It can be installed using any Python package manager, such as pip
or the more recent pipenv
. The following command will install the codepost
Python library on your entire system: Do so it will require you to have administrative privileges (this is why it is called with the prefix command sudo
) and to enter your login password.
sudo pip install codepost
If you receive a permission error, this means you do not have permission to make a system-wide installation of this package. You can instead install it in your own user account:
pip install --user codepost
2. Obtaining your codePost API key
To log into codePost, you are asked to provide your username and password. This helps codePost confirm who you are, and determine what you should have access to.
Perhaps, like the 39% of adult respondents to this 2017 Pew Survey on Americans and Cybersecurity, you use "the same or very similar" credentials across other sites. For that and many other reasons, it is inconvenient to store your actual password in a script, so we instead have you generate an API key, which is a single long password that will give you access to the API. If ever this key is compromised, it is easy to discard it and generate a new one.
To do so, log into codePost and go to the codePost user settings page and scroll down to the API token subsection, where you can copy-paste, reveal or regenerate a new API token.
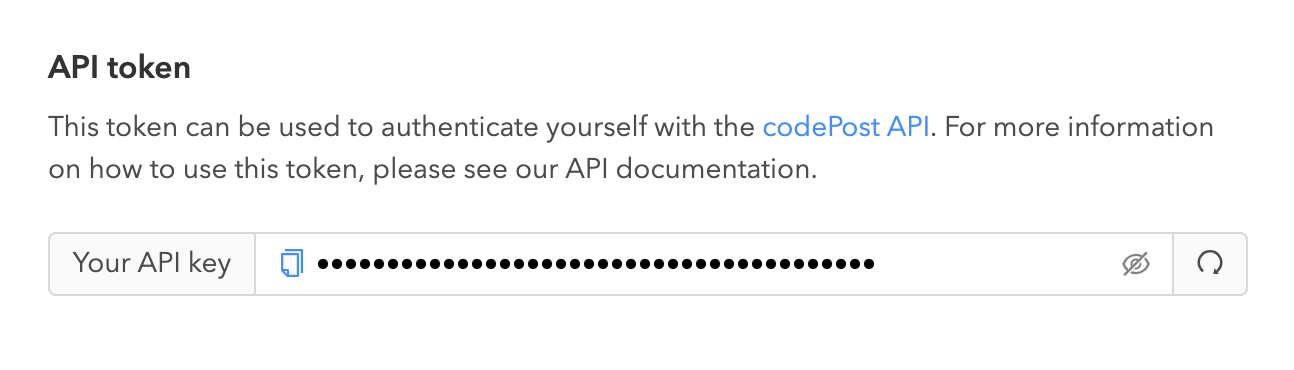
3. Testing the installation
With your API key, you can now open a Python terminal, to start experimenting. In the following three line snippet, first we load the codepost
Python SDK which we installed in Step 1, then we properly configure the API key which we retrieved in Step 2 to authenticate the calls made by the codePost Python SDK to the codePost API. Finally, we make a call to a method that will provide us a list of all the courses we have administrative access to:
import codepost
codepost.configure_api_key("aa9800f2-----------------------------912")
print(codepost.course.list_available())
Below we open a Python terminal and enter the above commands to see the output:
~$ python
Python 3.7.2 (default, Dec 30 2018, 08:55:50)
[Clang 10.0.0 (clang-1000.11.45.5)] on darwin
Type "help", "copyright", "credits" or "license" for more information.
>>> import codepost
>>> codepost.configure_api_key("aa9800f2-----------------------------912")
'aa9800f2-----------------------------912'
>>> print(codepost.course.list_available())
[{'id': 81, 'name': 'codePost demo course', 'period': 'DEMO', 'assignments': [298, 299], 'sections': [219, 220, 221], 'sendReleasedSubmissionsToBack': False, 'showStudentsStatistics': False, 'timezone': 'US/Eastern', 'emailNewUsers': False, 'anonymousGradingDefault': False, 'allowGradersToEditRubric': False}]
Our API key correctly authenticated us, and the call to the function codepost.course.list_available()
returned the codePost demo course
that we created when signing up. There is more detail on the list_available()
function in our API documentation.
Some notes on possible troubleshooting:
- If you get an
ImportError
when you try toimport codepost
: It seems the installation of thecodepost
Python SDK was either unsuccessful, or if you have multiple different versions of Python on your computer, it may have installed for the wrong version. - If you get an
AuthenticationAPIError
error when callingcodepost.course.list_available()
it may be that your API key is invalid or was not copy-pasted properly. - If you encounter any other issue, please email us at [email protected]!
Best practices for configuration
In the above example, we called the method codepost.configure_api_key
with the API key that we had fetched from the codePost user settings page.
This has the unfortunate consequence of hardcoding our API key in our script. This may not be desirable for security reasons, or because you may want to share your script with others, and it would be a hassle to edit the API key inside the script and make sure that your API key is not communicated to third parties.
For this reason, we provide two additional methods to setup your API key without having to hard-code it in your scripts: With a configuration file or an environment variable.
Using a codePost configuration file
The easiest possibility is to create a configuration file. This file can be called codepost-config.yaml
or .codepost-config.yaml
(the dot in front of the file means that the file will be hidden from view; these files are called dotfiles) and can be located either in the same directory as your script, or in your home directory.
This configuration file is a YAML file. YAML stands for "Yet Another Markup Language" and is essentially a list of key value pairs, formatted as key: value
, one per line. The simplest codePost configuration file contains only an API key under the name api_key
(please note the underscore):
api_key: aa9800f2-----------------------------912
Once this file is created and saved, and placed in the correct location, it will be automatically detected and used when importing the codePost Python SDK, and you will not need to call codepost.configure_api_key
.
The configuration file is very versatile and can be used to store more information. For instance, you could store your course name and course period (which you may reference regularly throughout your script) in a configuration file. That way, if you decide to share your script, whoever uses it will be able to customize the configuration file—but not have to edit the source code.
api_key: aa9800f2-----------------------------912
course_name: CS101
course_period: F2019
You can then access this configuration file as a dictionary using the function codepost.util.read_config_file()
. For instance, in a Python shell:
~$ python
Python 3.7.2 (default, Dec 30 2018, 08:55:50)
[Clang 10.0.0 (clang-1000.11.45.5)] on darwin
Type "help", "copyright", "credits" or "license" for more information.
>>> import codepost
>>> codepost.util.read_config_file()
{'api_key': 'aa9800f2fc896500206dbb48579bf15a31f96912', 'course_name': 'CS101', 'course_period': 'F2019'}
>>> config = codepost.util.read_config_file()
>>> print(config.get("course_name"))
CS101
Using an environment variable
You can also provide the API key through an environment variable called CP_API_KEY
. You can read more on how to set an environment variable on your operating system here.
Next steps
Now that you are able to use the codePost Python SDK, you are ready to follow with one of our tutorials.
Updated over 5 years ago